Projects
Miscellaneous programming projects outside of games that I have been working on.
Source code for select projects is available on request.
Conway's Game of Life
(C++)
Conway's Game of Life is a cellular automation designed to be a 0 player game, and instead relies on each cell's neighbors and an existing set of rules to determine if a cell lives or dies.
This project was made in C++ utilizing the wxWidgets library for UI interactions. This project improved my algorithmic thinking, understanding of data structures, memory management, and object oriented programming skills.
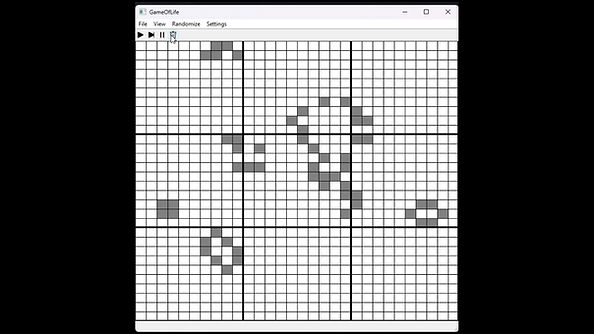
Calculator
(C++)
Calculator's are a staple of development portfolios for a reason.
This project was made in C++ utilizing the wxWidgets library for UI interactions. During this project I improved my understanding of how to parse user input effectively using delimiters, as well as how to work with singleton patterns.

Custom 3D Renderer
(C++)
A custom 3D rendering software from scratch that includes camera controls, lighting, and textures.
This project was made from scratch in C++ using the Gateware 3D math library, and a pre-existing software that allows you to modify the rgb values of an array, representing the pixels within a window.
Stage 1
This was the first week of development, in which basic rasterization and line drawing algorithms were implemented.

Stage 2
The second week of development included adding 3 dimensional verteces, as well as utilizing a world matrix for rotation, and a projection matrix to offset the "camera" at an angle. You can see that the geometry of a three dimensional shape is there, but not quite being represented as you would expect.
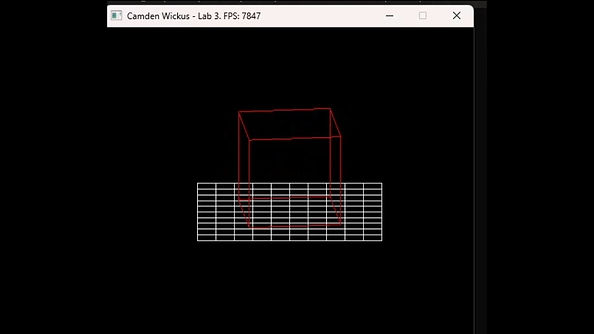
Stage 3
The third week of development included implementing a view matrix, which finalizes the 3D look and feel of the renderer this far. Now we can see the depth of the grid, and the cube truly looks like its rotating instead of just lines passing through each other. Additionally, this version uses depth buffering, along with texture mapping, to allow for clearly defined faces as well as rendered textures on the face of the cube.
Stage 4
The final week of development, I used the renderer to display a premade model of stonehenge, along with a starry night sky, skybox, and camera controls.

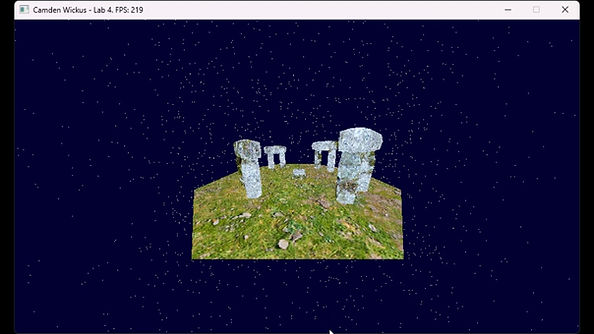
DX11 Data-Oriented Renderer
(C++) (HLSL)
A renderer software that uses DirectX11 and can render scenes imported from Blender.
This project uses the DirectX11 API, and utilizes the computer's GPU hardware instead of running solely on software like my custom renderer did. It also utlitizes functions of Blender, Renderdoc, CMake, and High Level Shader Language.
Stage 1
The first week of development was learning how to package information to send to the GPU rather than doing everything in software myself, as well as implementing fps controls and constructing some basic shapes to render.
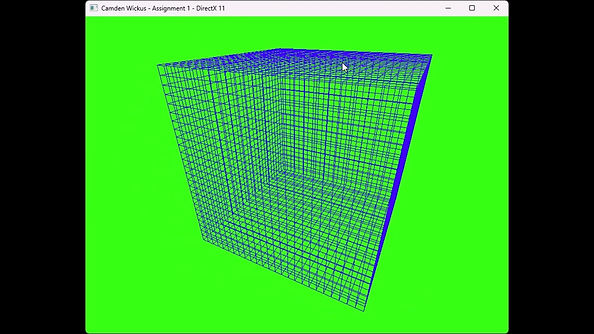
Stage 2
The second week, I used pre-made 3d model data to render out this Full Sail University logo, as well as implementing logic to get part of it to spin. You can also see reflections and shadows in this model, which use normal values and point lighting in HLSL to calculate brightness on any given pixel.
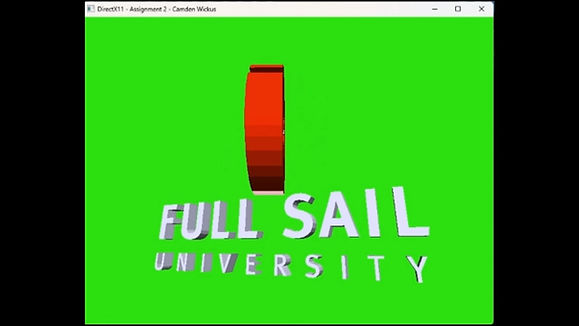
Stage 3
The third and fourth week of development, I used a pre-made python script that took a scene in Blender and output a data set, which could then be fed into the DX11 renderer and display the contents. I also implemented logic to switch between mutliple different scenes, and had a separate render camera for a minimap in the top left corner.

Data-oriented vs. Object Oriented
During the development of this renderer, I had to choose between using data-oriented rendering logic (faster but more complicated) or object-oriented (slower but easier to program). I chose to challenge myself and use data-oriented logic. Here is a look at the function that makes data-oriented rendering possible. As you can tell, it relies on indexing data from various arrays of information rather than making everything an object.
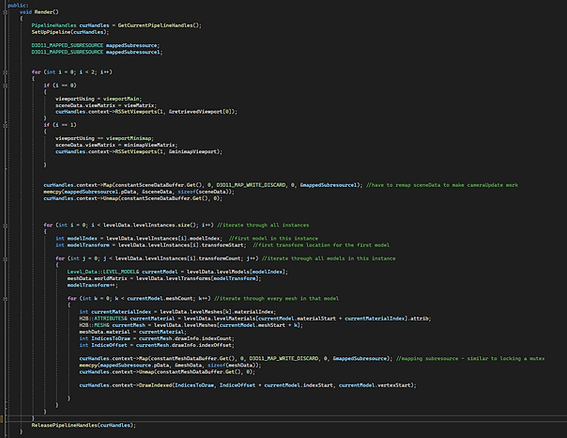
IPV4 Chat Server Using Winsock
(C++)
A chat server written in C++ that uses Winsock to establish a TCP connection between a host and client, allowing clients to register, login, and send messages to one another.
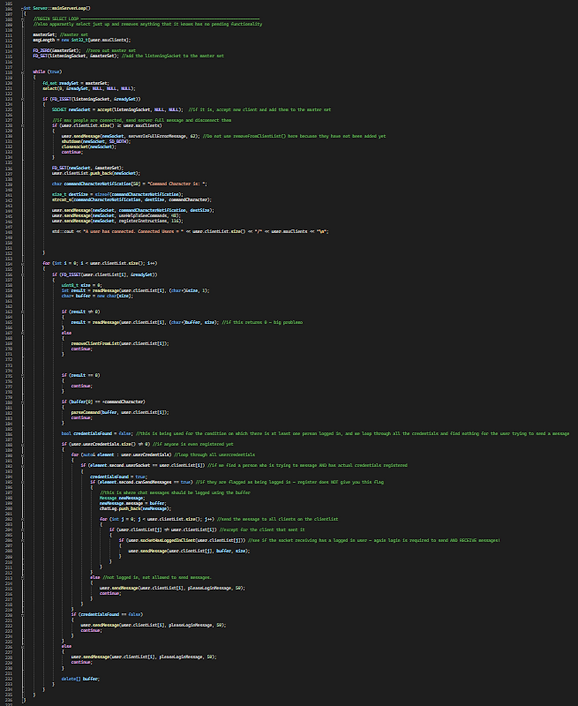
Pictured here is the main server loop, which utilizes the winsock Select function to wait for activity on the listening socket and connecting clients via a TCP handshake. A separate cpp then handles parsing client registration, communications, and error checking.
The image below shows 4 clients interacting with the server at once, as well as the wireshark capture confirming the TCP handshake and packets being sent.
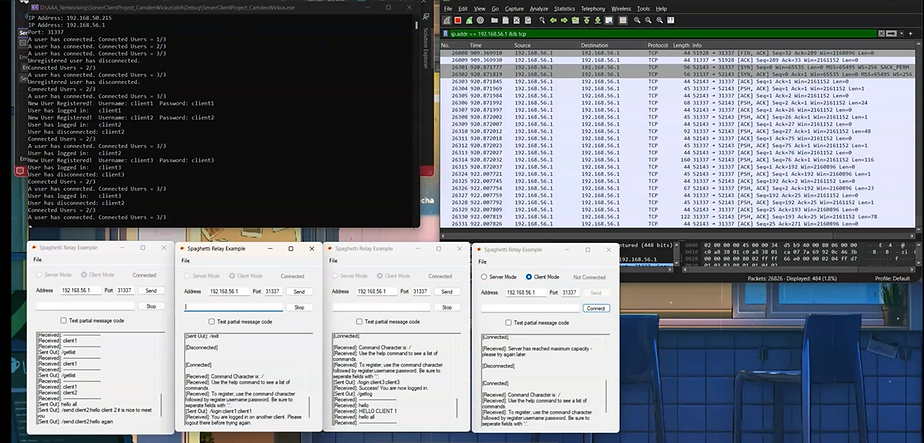
A more detailed look at the chat server and its functions can be found here: